In this post, a simple GDI+ function is presented to Scale images. In this function an image and the required scale ratio is sent a scaled image is returned. See the examples below:
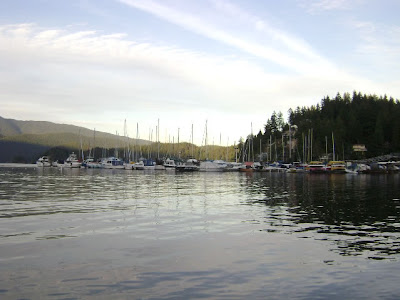
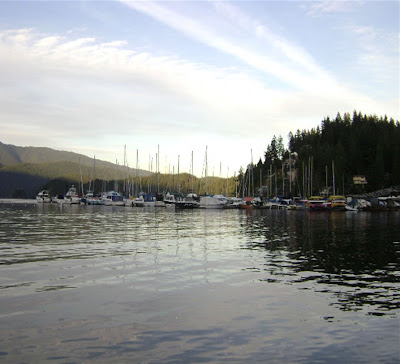
Here is the function:
////////////////////////////////////////////////////////////
//
// Description: A simple GDI+ function for scaling images
// Author: G. R. Roosta
// [royalty-free][digital][picture][photo][print]@
//
// License: Free To Use (No Restriction)
//
////////////////////////////////////////////////////////////
using System.Drawing;
using System.Drawing.Imaging;
using System.Drawing.Drawing2D;
using System;
namespace FABRICLAKE.INTELLIPHOTO.GDI.BASIC
{
public partial class Transform
{
public static Bitmap Scale(Bitmap bmp, double scaleWidth, double scaleHeight)
{
int oldWidth = bmp.Width;
int oldHeight = bmp.Height;
int oldX = 0;
int oldY = 0;
int newX = 0;
int newY = 0;
int newWidth = Convert.ToInt32(oldWidth * scaleWidth);
int newHeight = Convert.ToInt32(oldHeight * scaleHeight);
Bitmap scaledBMP = new Bitmap(newWidth, newHeight, bmp.PixelFormat);
scaledBMP.SetResolution(bmp.HorizontalResolution, bmp.VerticalResolution);
Graphics carpetlake;
try
{
carpetlake = Graphics.FromImage(scaledBMP);
}
catch
{
scaledBMP = new Bitmap(newWidth, newHeight, PixelFormat.Format24bppRgb);
scaledBMP.SetResolution(bmp.HorizontalResolution, bmp.VerticalResolution);
carpetlake = Graphics.FromImage(scaledBMP);
}
carpetlake.InterpolationMode = InterpolationMode.HighQualityBicubic;
carpetlake.DrawImage(bmp,
new Rectangle(newX, newY, newWidth, newHeight),
new Rectangle(oldX, oldY, oldWidth, oldHeight),
GraphicsUnit.Pixel);
carpetlake.Dispose();
return scaledBMP;
}
}
}
//
////////////////////////////////////////////////////////////
using System.Drawing;
using System.Drawing.Imaging;
using System.Drawing.Drawing2D;
using System;
namespace FABRICLAKE.INTELLIPHOTO.GDI.BASIC
{
public partial class Transform
{
public static Bitmap Scale(Bitmap bmp, double scaleWidth, double scaleHeight)
{
int oldWidth = bmp.Width;
int oldHeight = bmp.Height;
int oldX = 0;
int oldY = 0;
int newX = 0;
int newY = 0;
int newWidth = Convert.ToInt32(oldWidth * scaleWidth);
int newHeight = Convert.ToInt32(oldHeight * scaleHeight);
Bitmap scaledBMP = new Bitmap(newWidth, newHeight, bmp.PixelFormat);
scaledBMP.SetResolution(bmp.HorizontalResolution, bmp.VerticalResolution);
Graphics carpetlake;
try
{
carpetlake = Graphics.FromImage(scaledBMP);
}
catch
{
scaledBMP = new Bitmap(newWidth, newHeight, PixelFormat.Format24bppRgb);
scaledBMP.SetResolution(bmp.HorizontalResolution, bmp.VerticalResolution);
carpetlake = Graphics.FromImage(scaledBMP);
}
carpetlake.InterpolationMode = InterpolationMode.HighQualityBicubic;
carpetlake.DrawImage(bmp,
new Rectangle(newX, newY, newWidth, newHeight),
new Rectangle(oldX, oldY, oldWidth, oldHeight),
GraphicsUnit.Pixel);
carpetlake.Dispose();
return scaledBMP;
}
}
}
This comment has been removed by a blog administrator.
ReplyDelete